Today, I'm talking about EditText Floating Labels in Android application.
The new TextInputLayout
allows us to wrap EditText
view in order to display floating labels above the EditText
. This layout also let show error messages below the EditText
. When an EditText
has focus, the assigned hint will "float" above the view to the top-left hand side.
Firstly need to add library or add a dependency to gradle file.
dependencies {
compile 'com.android.support:design:22.2.0'
}
After it need to update the strings.xml file in the project.
<resources>
<string name="app_name">EditText Floating Labels Demo</string>
<string name="username">Username</string>
<string name="password">Password</string>
<string name="sign_in">Sign In</string>
<string name="login_error">Username can not be empty</string>
</resources>
Next step is updating layout for the project.
<?xml version="1.0" encoding="utf-8"?><br />
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingLeft="12dp"
android:paddingRight="12dp"
android:paddingTop="8dp"
android:paddingBottom="8dp">
<android.support.design.widget.TextInputLayout
android:id="@+id/login_layout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="8dp">
<EditText
android:id="@+id/edit_text_email"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:inputType="textEmailAddress"
android:hint="@string/username" />
</android.support.design.widget.TextInputLayout>
<android.support.design.widget.TextInputLayout
android:id="@+id/password_layout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="8dp">
<EditText
android:id="@+id/edit_text_password"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:inputType="textPassword"
android:hint="@string/password" />
</android.support.design.widget.TextInputLayout>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/sign_in"
android:id="@+id/sing_in_button"
android:layout_gravity="center_horizontal" />
</LinearLayout>
Last step is update activity.
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main_activity);
}
}
We can also show error in TextInputLayout
. We need to use method setErrorEnabled(boolean)
and setError(CharSequence)
. As example, we can check login field during focus another view. For it need to update onCreate
function and implement onFocusChange
function.
public class MainActivity extends AppCompatActivity implements View.OnFocusChangeListener {
TextInputLayout mUsernameLayout;
EditText mUsername;
EditText mPassword;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main_activity);
mUsernameLayout = (TextInputLayout) findViewById(R.id.login_layout);
mUsername = (EditText) findViewById(R.id.edit_text_email);
mPassword = (EditText) findViewById(R.id.edit_text_password);
mUsername.setOnFocusChangeListener(this);
mPassword.setOnFocusChangeListener(this);
}
@Override
public void onFocusChange(View v, boolean hasFocus) {
if (v != mUsername && mUsername.getText().toString().isEmpty()) {
mUsernameLayout.setErrorEnabled(true);
mUsernameLayout.setError(getResources().getString(R.string.login_error));
} else {
mUsernameLayout.setErrorEnabled(false);
}
}
}
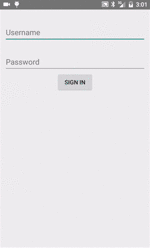