Today, I'm talking about integrating Google's "+1" button to the Android application. This allows users to share your application in "Google+".
Firstly, you need to add a dependency to the gradle file.
dependencies {
compile "com.google.android.gms:play-services:7.3.0"
}
Afterward, we need to add a "+1" button to your layout. A size of the PlusOneButton
button may be standard, tall, small or medium. We need to change the size
attribute for changing the size of the button.
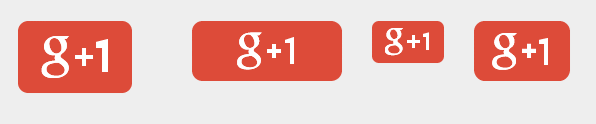
<com.google.android.gms.plus.PlusOneButton
xmlns:plus="http://schemas.android.com/apk/lib/com.google.android.gms.plus"
android:id="@+id/plus_one_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
plus:size="standard"
plus:annotation="none" />
The next step is updating an activity.
public class MainActivity extends AppCompatActivity {
private static final int PLUS_ONE_REQUEST_CODE = 0;
private static final String APP_URL = "https://play.google.com/store/apps/details?id=app_package";
private PlusOneButton mPlusOneButton;
private GoogleApiClient mGoogleApiClient;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_plus_one);
mPlusOneButton = (PlusOneButton) findViewById(R.id.plus_one_button);
mGoogleApiClient = new GoogleApiClient.Builder(this)
.addApi(Plus.API)
.addScope(Plus.SCOPE_PLUS_LOGIN)
.useDefaultAccount()
.build();
}
@Override
protected void onResume() {
super.onResume();
mPlusOneButton.initialize(APP_URL, PLUS_ONE_REQUEST_CODE);
}
@Override
protected void onStart() {
super.onStart();
mGoogleApiClient.connect();
}
@Override
protected void onStop() {
super.onStop();
mGoogleApiClient.disconnect();
}
}
The last step is to change the app_package
to your application package.
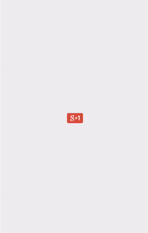