Introduction
I recently figured out that after migrating my blog to a new platform, some of my articles have a new URL while some of them weren't available. As a result, some users have seen the "Page not found" message during the last few months. Thank you, analytics, for sharing this data with me.
I decided to create a small test case to verify that the redirect functionality works well for pages with updated URL addresses.
My main idea was to check the old URL of the page, the new URL of the page, and the title of the article. I decided to go with a Cypress framework which allows me to create end-to-end (E2E) test cases. Another benefit is that we can create many test cases based on the array or JSON file. This approach can be beneficial if you want to check redirect and use platforms like WordPress, Ghost, etc.
In this article, I want to share what Cypress is, and explain how to check redirects with it, and verify the article's title.
What is Cypress?
Cypress is a front-end testing framework that easily writes tests for web applications.
Cypress is waiting for loading data without additional configuration. This feature makes this framework suitable for our case.
Adding Cypress to the NPM project
We can use Cypress by adding a dependency to the NPM project and adding test cases.
To create an NPM project by CLI (Command-line interface), you need to install Node.js to your machine. I recommend installing NVM (Node Version Manager) for the possibility of changing the version of Node.js from the terminal.
To create an NPM project, you can use the following command and enter all required data.
npm init
The next step is to add a Cypress dependency. This dependency can be added as "dev dependency", meaning such dependencies are unneeded for production.
npm install cypress --save-dev
Afterward, we can open a Cypress application.
npx cypress open
When the app is open, you can see a list of folders and files from the "cypress/integration" directory. By default, Cypress generates examples of test cases. You can run and explore them for a better understanding of the possibility of the framework.
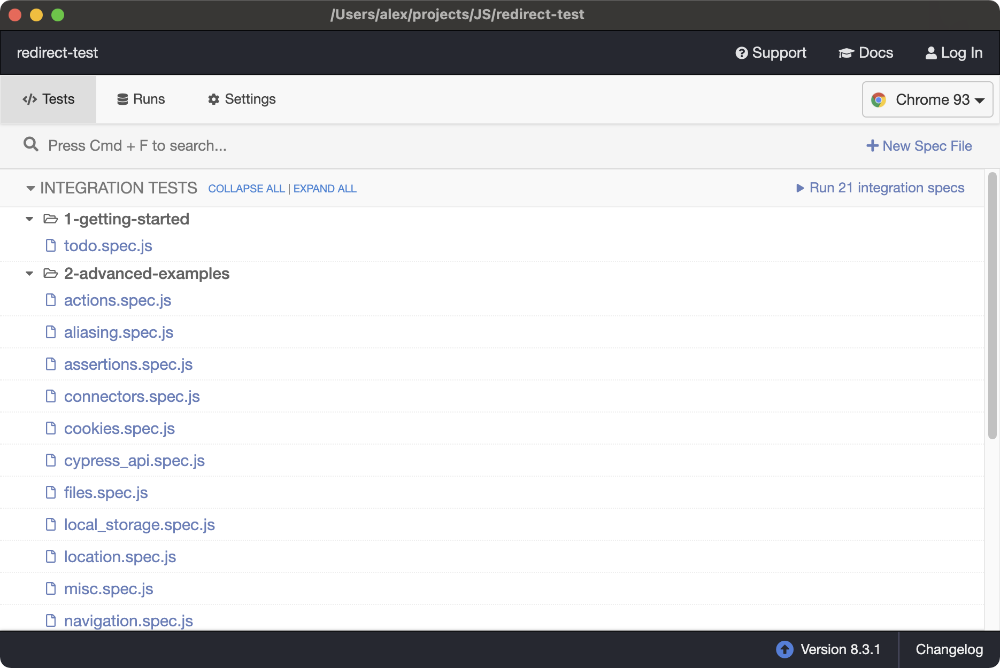
You can read more about the features of Cypress here.
Verification of a redirect functionality with Cypress
I want to create a test case for the verification redirect functionality for a specific website. A test case should redirect from an old URL to a new one and check the article's title on the page.
I want to use the following object structure for testing the redirect functionality. Later on, we will read these data from a JSON file.
{
"title": "TITLE OF THE ARTICLE",
"from": "OLD URL",
"to": "NEW URL"
}
So, let's create a "website-redirect" folder in the "cypress/integration" one and add a test case to it. This test case verifies the redirect functionality.
describe("website redirects", () => {
it("webpage redirect", () => {
const page = {
"title": "Android testing: Espresso (Part 3)",
"from": "http://alexzh.com/tutorials/android-testing-espresso-part-3/",
"to": "https://alexzh.com/android-testing-espresso-part-3/"
}
cy.visit(page.from, { failOnStatusCode: false });
cy.url()
.should("be.equals", page.to)
cy.title()
.should("include", page.title);
});
});
The cy.visit(page.from)
opens the page with a URL from the page.from
value. We can use the options parameter which can help us configure the additional option for loading the page in the browser. The failOnStatusCode: false
wouldn't fail the test if the response code is different than "2XX" or "3XX".
The cy.url()
retrieves the current URL of the page, so we can verify whether the URL was updated after the redirect.
The cy.title()
gets the document.title
property of the active page.
This approach works well, but we have a page object in a test case. To run tests for all pages, let's move this page object to a separate JSON file which can act as input for the tests. The predefined structure of the "cypress" folder already has the "fixtures" directory, which is usually used for loading a set of data located in a file. It's a perfect place for our redirect-data.json
file.
[
{
"title": "Android testing: Espresso (Part 3)",
"from": "http://alexzh.com/tutorials/android-testing-espresso-part-3/",
"to": "https://alexzh.com/android-testing-espresso-part-3/"
},
{
"title": "Guide: Testing Android Notifications",
"from": "http://alexzh.com/tutorials/guide-testing-android-notifications/",
"to": "https://alexzh.com/guide-testing-android-notifications/"
},
...
]
When a JSON file is created, we can load an array for our test cases in a few different ways:
- The
cy.fixture(filePath)
loads a fixed set of data located in a file. This option can be used inside the test case. You can read more in the documentation. - The
require/import
allows to load a JSON file and use data outside of the test case.
The second option is a better option for us because we can create multiple tests based on an array of objects from the JSON file In this case, we can use the page's name for the name of a test case.
import data from '../../fixtures/redirect-data.json';
describe("website redirects", () => {
data.forEach(pageObj => {
it(`redirect of "${pageObj.title}" page`, () => {
cy.visit(pageObj.from, {failOnStatusCode: false});
cy.url()
.should("be.equals", pageObj.to)
cy.title()
.should("include", page.title);
});
});
});
When we have multiple values in the array, our report will use the titles of pages.
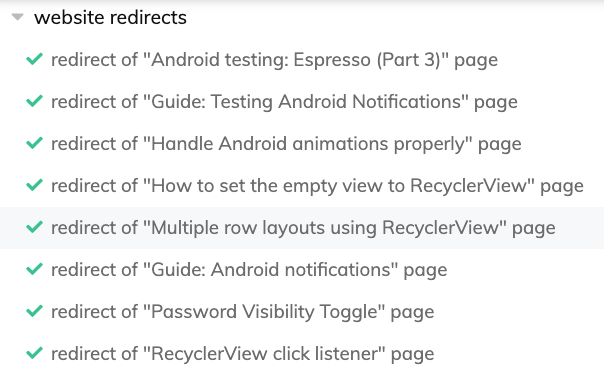
Summary
Many websites often use the redirect functionality. The test automation allows us to simplify the routine operations such as redirect verification, search an article, newsletter signup, etc.
The Cypress is one of many frameworks that helps us to carry out such verifications. In some cases, it's not the optimal solution because you need to launch a browser and implement end-to-end verification. However, if you use CMS like WordPress, Ghost, and many others, it can be a fast and straightforward solution to verify that all redirects work correctly.
You can find the source code in this article here.
Thank you, Varun A P, for reviewing the article.