Introduction
The "Regional Preferences" settings screen appeared in Android 14, and it allows users to set a few regional preferences:
- First Day of Week
- Temperature Unit
This feature is convenient for people who are used to one temperature unit, but live in the country where another one is used. Many applications have their own preferences, and users can set temperature units, the first day of the week, and hours cycle preferences for every app.
To find the Regional Preferences setting, go to Settings > System > Languages and press the Regional Preferences menu item.
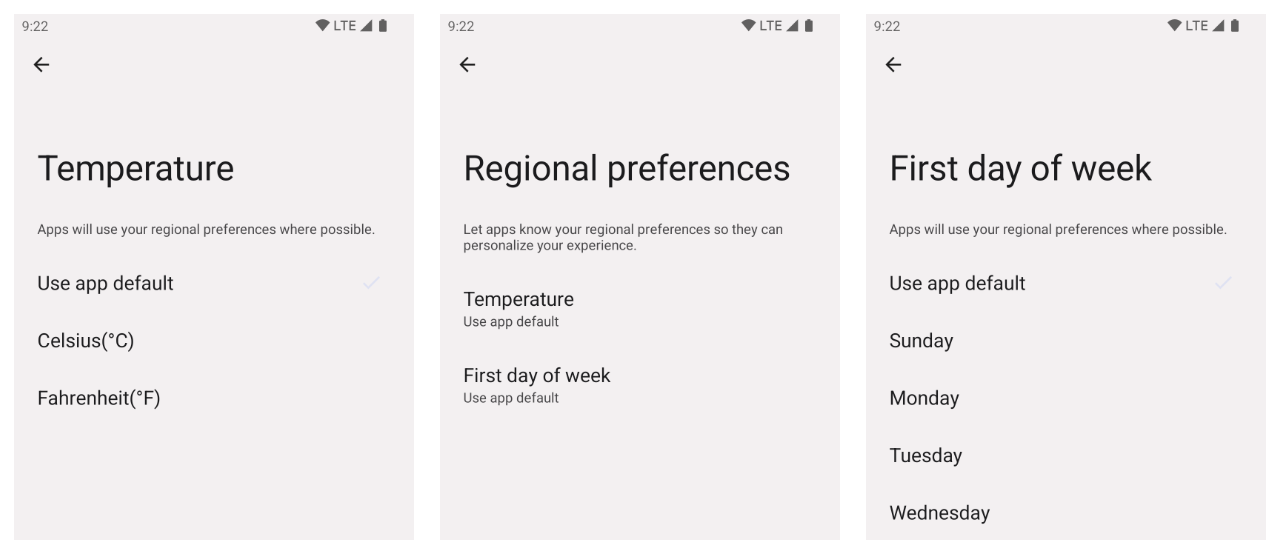
Getting Regional Preference Values
We can use the following data by using new LocalePreferences
API:
- The temperature units
- The first day of the week
- The hour cycle
- The calendar type
To use the API to get this data, we need to use the androidx.core:core-ktx
dependency with version 1.12.0-alpha01
or higher.
You can find information about all versions of "androidx.core:core-ktx" dependency here.
dependencies {
...
implementation "androidx.core:core:1.12.0-rc01"
}
Getting the temperature units
The LocalePreferences.getTemperatureUnit()
returns a String
value which represents a selected temperature unit:
- "celsius" (°C)
- "fahrenhe" (°F)
We have more functions for getting the temperature units for a specific locale:
getTemperatureUnit(Locale locale)
getTemperatureUnit(Locale locale, boolean resolved)
You can also compare these values with a specific temperature unit by using constants (LocalePreferences.TemperatureUnit.FAHRENHEIT
).
You can find all the constants for the TemperatureUnit
class here.
Getting the first day of the week
The LocalePreferences.getFirstDayOfWeek()
returns a String
value which represents a selected day of the week:
- "sun" - Sunday
- "mon" - Monday
- "tue" - Tuesday
- "wed" - Wednesday
- "thu" - Thursday
- "fri" - Friday
- "sat" - Saturday
We have more functions to for getting the first day of the week for a specific locale:
getFirstDayOfWeek(Locale locale)
getFirstDayOfWeek(Locale locale, boolean resolved)
You can also compare these values with a specific day of the week by using constants (LocalePreferences.FirstDayOfWeek.MONDAY
).
You can find all the constants for the FirstDayOfWeek
class here.
Getting the hour cycle
The LocalePreferences.getHourCycle()
returns a String
value which represents the hour cycle for the current Locale
. It returns the following values:
- "h11" - 12 Hour System (0-11)
- "h12" - 12 Hour System (1-12)
- "h23" - 24 Hour System (0-23)
- "h24" - 24 Hour System (1-24)
We have more functions to for getting the hour cycle for a specific locale:
getHourCycle(Locale locale)
getHourCycle(Locale locale, boolean resolved)
You can also compare these values with a specific hour cycle by using constants (LocalePreferences.HourCycle.H24
).
You can find all the constants for the HourCycle
class here.
Getting the calendar type
The LocalePreferences.getCalendarType()
returns a String
value which represents the calendar type for the current locale. It returns the following values:
- "chinese" - Chinese Calendar
- "dangi" - Dangi Calendar (Korea Calendar)
- "gregorian" - Gregorian Calendar
- "hebrew" - Hebrew Calendar
- "indian" - Indian National Calendar
- "islamic" - Islamic Calendar
- "islamic-civil" - Islamic Calendar (tabular, civil epoch)
- "islamic-rgsa" - Islamic Calendar (Saudi Arabia, sighting)
- "islamic-tbla" - Islamic Calendar (tabular, astronomical epoch)
- "islamic-umalqura" - Islamic Calendar (Umm al-Qura)
- "persian" - Persian Calendar
We have more functions for getting the calendar type for a specific locale:
getCalendarType(Locale locale)
getCalendarType(Locale locale, boolean resolved)
You can also compare these values with a specific calendar type by using constants (LocalePreferences.CalendarType.CHINESE
).
You can find all the constants for the CalendarType
class here.
Example and Use Cases
One of the simplest ways to compare values between different locales is to create a BroadcastReceiver
which logs new values for a selected locale after changing it in the Settings > System > Languages > System Languages screen.
class LocaleReceiver : BroadcastReceiver() {
@RequiresApi(Build.VERSION_CODES.TIRAMISU)
override fun onReceive(context: Context, intent: Intent) {
val localePreferenceData = """
getTemperatureUnit: ${LocalePreferences.getTemperatureUnit()}
getFirstDayOfWeek: ${LocalePreferences.getFirstDayOfWeek()}
getHourCycle: ${LocalePreferences.getHourCycle()}
getCalendarType: ${LocalePreferences.getCalendarType()}
""".trimIndent()
Log.d("New locale prefs values", localePreferenceData)
}
}
The next step is to register the LocaleReceiver
receiver in the AndroidManifest.xml
file:
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
...
<application
...>
<receiver
android:name=".LocaleReceiver"
android:enabled="true"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.LOCALE_CHANGED" />
</intent-filter>
</receiver>
...
</application>
</manifest>
Let's compare values for the "English (United States)" and "English (Netherlands)" locales.
English (United States) | English (Netherlands) | |
---|---|---|
Temperature Unit | fahrenhe | celsius |
First Day of Week | sun | mon |
Hour Cycle | h12 | h23 |
Calendar Type | gregorian | gregorian |
Let's take a look at a few use cases when this data can be helpful.
Temperature Unit
Many applications show weather data and they usually have their own settings for selecting preferred temperature units. Default data for this screen can be based on the values from the "Regional Preferences" screen.
The date in the application can be converted to a specific temperature unit based on user preferences from the "Regional Preferences" screen.
First Day of Week
The "DatePicker" component is used in many applications for selecting the date and we can decide if we want to start the week in this component from Saturday or Monday. When we get information about the first day of the week, we can make a better experience for the user.
Hour Cycle
You can find time in many applications. Usually, we see a specific time when a user creates a note, pays for a product, or checks information about delivery time. All data can have benefits from using the hour cycle preference and time will be formatted according to user preferences.
Conclusion
The "Regional Preferences" settings screens appeared in Android 14 and developers can start using API to get temperature units and the first day of the week.
We can get user preferences for temperature units and first day of week using the following functions:
LocalePreferences.getTemperatureUnit()
LocalePreferences.getFirstDayOfWeek()
In addition to that, we can get information about hour cycle and calendar type from the current locale:
LocalePreferences.getHourCycle()
LocalePreferences.getCalendarType()
I have doubts that this API will be widely used in the nearest future because many apps already have their own built-in implementation of configuring these properties in app settings and it works with all Android OS versions. However, I think that this API will be used in the future.