Today I'm talking about material style for dialogs in Android application.
STEP 1: Add libraries to the project
Firstly need to add libraries to gradle file for a project.
dependencies {
compile fileTree(dir: 'libs', include: ['*.jar'])
compile 'com.android.support:appcompat-v7:23.0.1'
}
STEP 2: Create dialog in application
For creating dialog in application you can use AlertDialog
component. The best way way is to use android.support.v7.app.AlertDialog
.
Next snippet of code show how you can create a dialog:
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setTitle(R.string.info_title);
builder.setMessage(R.string.info_description);
builder.setPositiveButton(R.string.ok, null);
builder.setNegativeButton(R.string.cancel, null);
builder.show();
In this example we don't use click action for dialog, if you want do it you must change null
to
new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
//SOME CODE
}
}
This source code use resources from string file.
STEP 3: Add strings to the application
The best way is to use string resources instead of strings in source code. It's better for supporting multiple languages. We need to open file strings.xml
from res/values
folder.
<resources>
<string name="ok">OK</string>
<string name="cancel">Cancel</string>
<string name="info_title">Information</string>
<string name="info_description">Android is a mobile operating system (OS) currently developed by Google, based on the Linux kernel and designed primarily for touchscreen mobile devices such as smartphones and tablets.
From Wikipedia</string>
</resources>
After these steps you can run the application and check dialog.
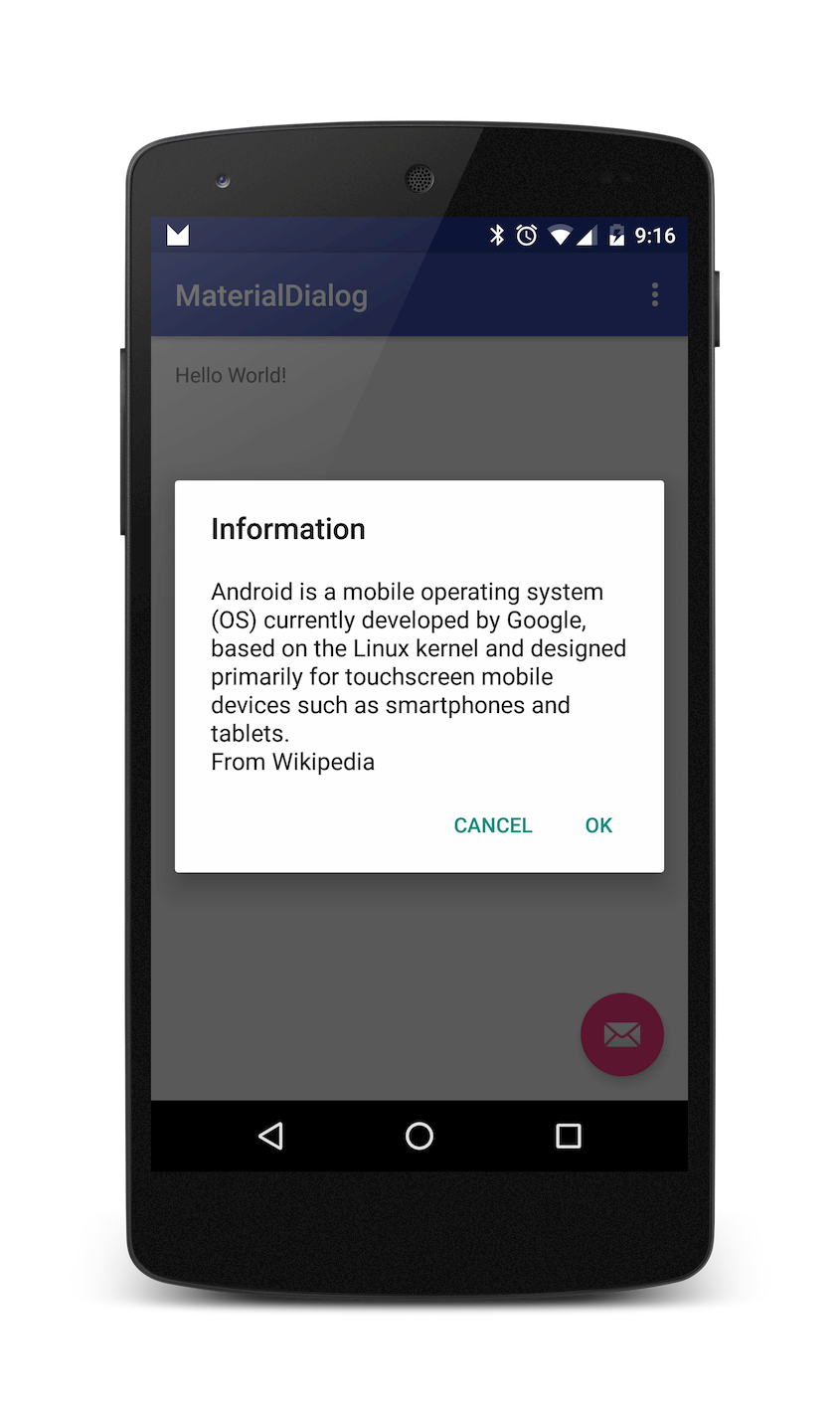
STEP 4: Create style for Dialog and apply this style for the application
We need to open file styles.xml
from res/values
folder and add style.
<style name="AppCompatAlertDialogStyle" parent="Theme.AppCompat.Light.Dialog.Alert">
<item name="colorAccent">#546E7A</item>
<item name="android:textColorPrimary">#37474F</item>
<item name="android:background">#B0BEC5</item>
</style>
- colorAccent - color of buttons
- textColorPrimary - color of text
- background - color of background of dialog
You can set style just for dialog or for all dialogs in application.
- If you want to set style for dialog you can setup it during creating of
AlertDialog.Builder
. We need to change this line:
AlertDialog.Builder builder = new AlertDialog.Builder(this);
to
AlertDialog.Builder builder = new AlertDialog.Builder(this, R.style.AppCompatAlertDialogStyle);
In this case AppCompatAlertDialogStyle
it's a name of style.
- If you want add some style for all dialogs in your application you can add your style to application style, as example:
<style name="AppTheme" parent="Theme.AppCompat.Light.NoActionBar">
<item name="colorPrimary">@color/colorPrimary</item>
<item name="colorPrimaryDark">@color/colorPrimaryDark</item>
<item name="colorAccent">@color/colorAccent</item>
<item name="alertDialogTheme">@style/AppCompatAlertDialogStyle</item>
</style>
<style name="AppCompatAlertDialogStyle" parent="Theme.AppCompat.Light.Dialog.Alert">
<item name="colorAccent">#546E7A</item>
<item name="android:textColorPrimary">#37474F</item>
<item name="android:background">#B0BEC5</item>
</style>
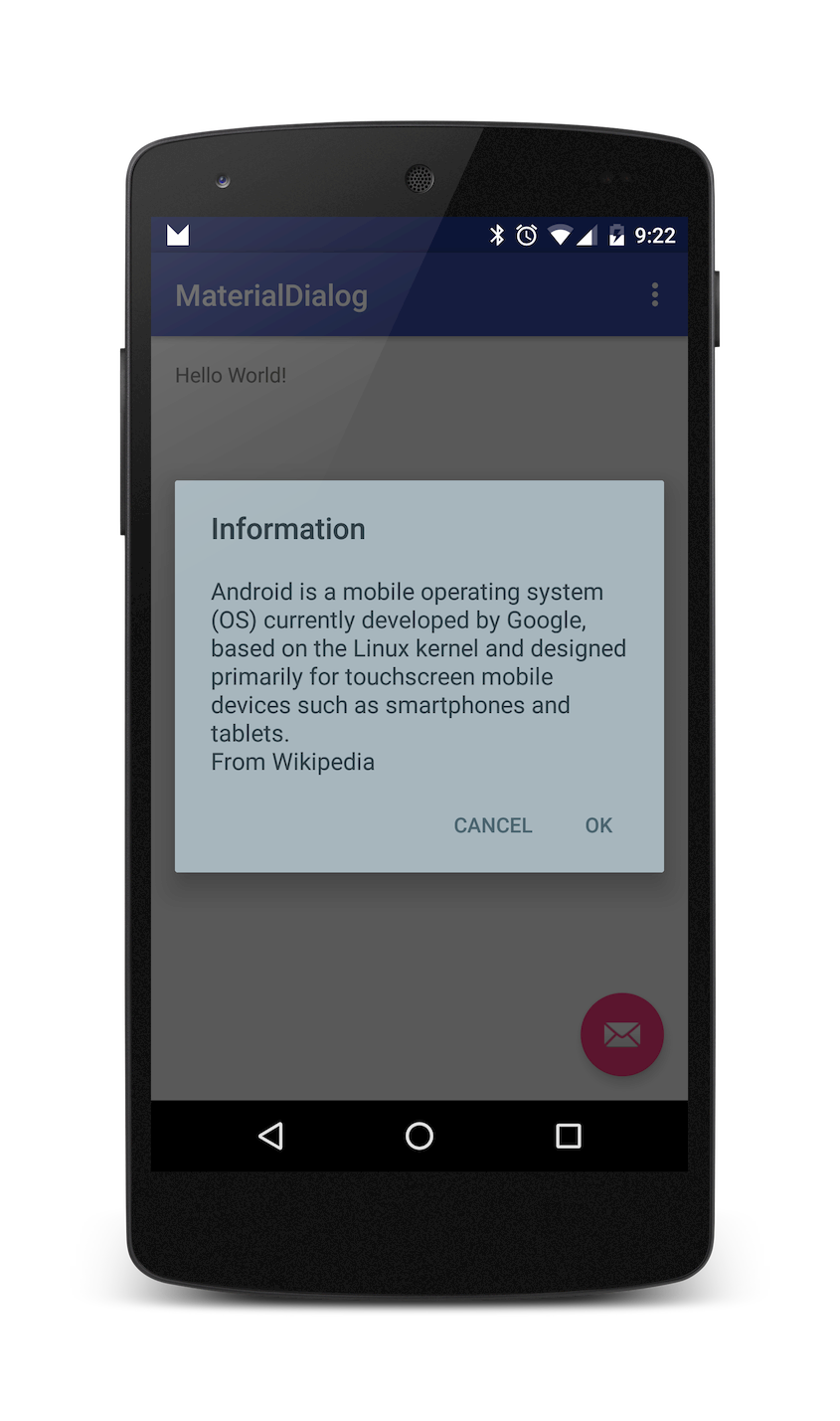